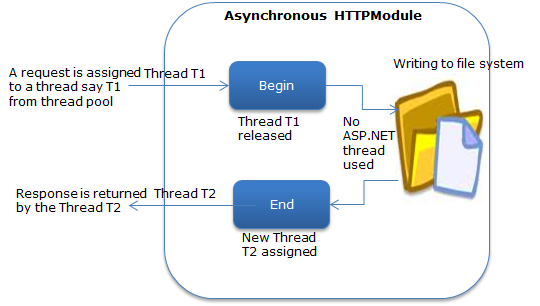
http://brijbhushan.net/2012/04/24/writing-asynchronous-http-module-in-asp-net-4-5/
public
string
HomeMetaTags()
{
System.Text.StringBuilder strMetaTag =
new
System.Text.StringBuilder();
strMetaTag.AppendFormat(
@"<meta content='{0}' name='Keywords'/>"
,
"Home Action Keyword"
);
strMetaTag.AppendFormat(
@"<meta content='{0}' name='Descption'/>"
,
"Home Description Keyword"
);
return
strMetaTag.ToString();
}
Reminder: Don't use web.config to restrict access, use [Authorize]
[AllowAnonymous]
public
ActionResult Login()
class
HomeController : AuthorizedController
<modules runAllManagedModulesForAllRequests="true" />
input:required{ border-color:red; } input:optional{ border-color:whitesmoke; }
<input type="text" name="name" id="first-name" placeholder="enter you first name" required />
public class when_a_new_user_registers_with_invalid_data : SpecsFor<MvcWebApp> { protected override void Given() { SUT.NavigateTo<AccountController>(c => c.Register()); } protected override void When() { SUT.FindFormFor<RegisterModel>() .Field(m => m.Email).SetValueTo("notanemail") //.Field(m => m.UserName).SetValueTo("Test User") --Omit a required field. .Field(m => m.Password).SetValueTo("P@ssword!") .Field(m => m.ConfirmPassword).SetValueTo("SomethingElse") .Submit(); } //...snip... }